Relay is an electromagnetic switch, which generally use in industries and houses to control the different machine and appliances like a lamp, water pump, road light AC compressor..etc.
Relay is a combination of the mechanical switch and an electromagnet, it prefers when we want to control a device or machine using the low strength signal.
In this tutorial, we will learn about the interfacing of the relay (relay connection) with microcontroller and learn how to control a device using the relay.
Working of relay
Relay works on the basis of electromagnetic force. Internally relay consist of a coil of wire wrapped soft iron core. When we have applied the voltage between the ends of the wrapped wire, then there is a current flow in the wrapped wire. So due to this current, a magnetic field would be generated and attract the switch from NC (normal close) to NO (normal open).
Simple relay connection
In the below image, I am describing the relay connection with bulb. The gif image describing how we can bulb using the SPDT (single pole double throw) relay.
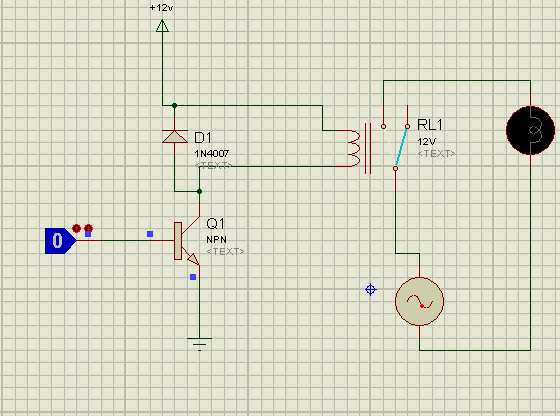
Sample program to Interface relay with 8051 microcontrollers.
In this program, we will control the bulb using serial communication to sends a specific command. When the user sends 1 (command) from the virtual window to the microcontroller, then bulb will be turned on and if the user sends 2 (command) from the virtual window then bulb will be turned off.
Note: here I have assumed, you have the knowledge of the serial communication.
#include <reg51.h> #include <string.h> #define LCD P1 /*Attached Lcd on Port*/ sbit rs = P2^4; /*Configure RS Pin*/ sbit rw = P2^5; /*Configure R/W pin*/ sbit e = P2^6; /*Configure Enable pin*/ sbit Relay = P2^0; //Attach Relay with P2.0 /*Function to write command on Lcd*/ void LcdCommand(const char cCommand); /*Function to display message on Lcd*/ void DisplayMessage(const char *pszMessage); /*Function To Initialize Lcd*/ void LcdInit(void); /*Function to Provide delay*/ void Delay(unsigned int); /*Main function*/ int main(void) { char acReceiveBuff[2]= {0}; //Store receive byte Relay = 0; //PIn make Output TMOD = 0X20; //configure timer in mode2 to generate baudrate SCON = 0X50; //SCON register-receive enabled,mode1 communication enabled TH1 = 0xFD; //timer1 in mode2 is used for baud rate generation RI = 0; //RI flag cleared SBUF = 0X00;//SBUF i.e. uart buffer initialized to 0 LcdInit(); //initialize the lcd LcdCommand(0x80); /*Address of DDRAM*/ DisplayMessage("Enter Choice:"); TR1=1; //Start the timer to generate baudrate while(1) //Infinite loop { LcdCommand(0x8d); /*Address of DDRAM*/ while(RI == 0); //Wait for receive acReceiveBuff[0] = SBUF; //Copy serial buffer resistor into buffer DisplayMessage(acReceiveBuff); RI = 0; //RI flag cleared if(!strncmp(acReceiveBuff,"1",1)) //Lamp on, when enter 1 { Relay = 1; } else if(!strncmp(acReceiveBuff,"2",1)) //Lamp off, when enter { Relay = 0; } else { memset(acReceiveBuff,0,sizeof(acReceiveBuff)); } } } /*Function to write command on Lcd*/ void LcdCommand(const char cCommand) { rs = 0; rw = 0; e = 1; LCD = cCommand; Delay(1); e=0; } /*Function to Display message on Lcd*/ void DisplayMessage(const char *pszMessage) { rs = 1; rw = 0; while(*pszMessage!='\0') { e = 1; LCD = *pszMessage; Delay(1); e=0; pszMessage++; } } /*Function to Provide Delay*/ void Delay(unsigned int i) { int j,k; for(j=0; j<i; j++) for(k=0; k<1275; k++); } /*Initialise the LCD*/ void LcdInit(void) { LcdCommand(0x01); Delay(2); LcdCommand(0x38); Delay(2); LcdCommand(0x06); Delay(2); LcdCommand(0x0c); Delay(2); }
Recommended Post:
- Led blinking program in c for 8051.
- Interfacing of switch and led using the 8051
- Moving message display on LCD using 8051
- LCD 4-bit mode c code for 8051.
- Create LCD custom characters for 16×2 alphanumeric LCD
- Interfacing of keypad with 8051
- Electronic digital lock using the 8051
- Interfacing of EEPROM with 8051 microcontrollers using I2C
- Embedded c interview questions.
- 8051 Microcontroller Pin Diagram and Pin Description.
- Can protocol interview questions.
- 8051 Architecture.
- Serial port programming using Win32.
Your opinion matters
Here I have tried to explain the about the relay and tried to explain the connection of relay with 8051 micro-controller through an NPN transistor. I would like to know your thought about the above-discussed topic, so please don’t forget to write a comment in a comment box.