In this blog post, we learn how to write a C program to find the largest and smallest element in the array? So here we will write the C program to find the smallest and largest element in an unsorted array. We will also see how to display the largest and smallest elements in an array using C programming.
Example,
Input: int arr[] = {3, 18, 10, 4, 2, 22, 150}; Output: Min = 2 , Max = 150
Logic to find the largest and smallest element in the array
So let’s see the logic to find the largest and smallest element in the array. Suppose arr is an integer array of size N (arr[N] ), the task is to write the C program to find largest and smallest element in the array.
1. Create two intermediate variables small and large.
2. Initialize the small and large variable with arr[0].
3. Now traverse the array iteratively and keep track of the smallest and largest element until the end of the array.
4. In the last you will get the smallest and largest number in the variable small and large respectively.
5. print both variables using the printf a library function.
If you want to learn more about the C language, you can check this course, Free Trial Available.
C Program to find largest and smallest element in array
#include <stdio.h> //Calculate array size #define ARRAY_SIZE(a) sizeof(a)/sizeof(a[0]) int main() { int arr[] = {3, 18, 10, 4, 2, 22, 150}; int i, small, large; const int N = ARRAY_SIZE(arr); small = arr[0];//Assume first element is smallest large = arr[0];//Assume first element is largest //iterate through the array for (i = 1; i < N; i++) { if (arr[i] < small) { small = arr[i]; } if (arr[i] > large) { large = arr[i]; } } printf("Largest element is : %d\n", large); printf("Smallest element is : %d\n", small); return 0; }
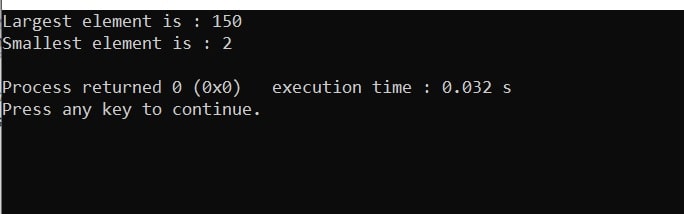
Recommended Articles for you:
- Best gift for programmers.
- Best electronic kits for programmers.
- Write C program to find the missing number in a given integer array of 1 to n
- C program to find the most popular element in an array
- C program to find even occurring elements in an array of limited range
- Find sum of all sub-array of a given array.
- C program to segregate even and odd numbers
- Find an element in array such that sum of left array is equal to sum of right array.
- C Program to find the count of even and odd elements in the array.
- Write C program to find the sum of array elements.
- C program to find odd occurring elements in an array of limited range
- Find the sum of array elements using recursion
- C Program to reverse the elements of an array
- C Program to find the maximum and minimum element in the array
- Calculate size of an array in without using sizeof in C
- How to create a dynamic array in C?
- How to access 2d array in C?
- Dangling, Void, Null and Wild Pointers
- Function pointer in c, a detailed guide
- Memory Layout in C.
- 100 C interview Questions
- File handling in C.